In the world of healthcare, building applications that handle sensitive patient data comes with a crucial requirement: compliance with the Health Insurance Portability and Accountability Act (HIPAA). This U.S. law establishes standards for protecting patient data, and ensuring privacy and security when dealing with health information. If you're building a healthcare app with Flutter, it's essential to make sure your app meets HIPAA compliance standards.
In this blog, we'll explore how to build a HIPAA-compliant healthcare app using Flutter and what key steps you need to follow to ensure your app adheres to these regulations.
What is HIPAA Compliance?
HIPAA is a federal law designed to protect patient health information (PHI). Any app that stores, processes, or transmits PHI must comply with HIPAA. Failing to do so can lead to serious legal consequences and hefty fines. HIPAA compliance is particularly critical in healthcare apps where data such as patient records, medical histories, or even video consultations are managed.
There are two main parts of HIPAA compliance:
- Privacy Rule: Regulates how PHI can be used and disclosed.
- Security Rule: Specifies safeguards to ensure the confidentiality, integrity, and availability of PHI, particularly when stored or transmitted electronically (ePHI).
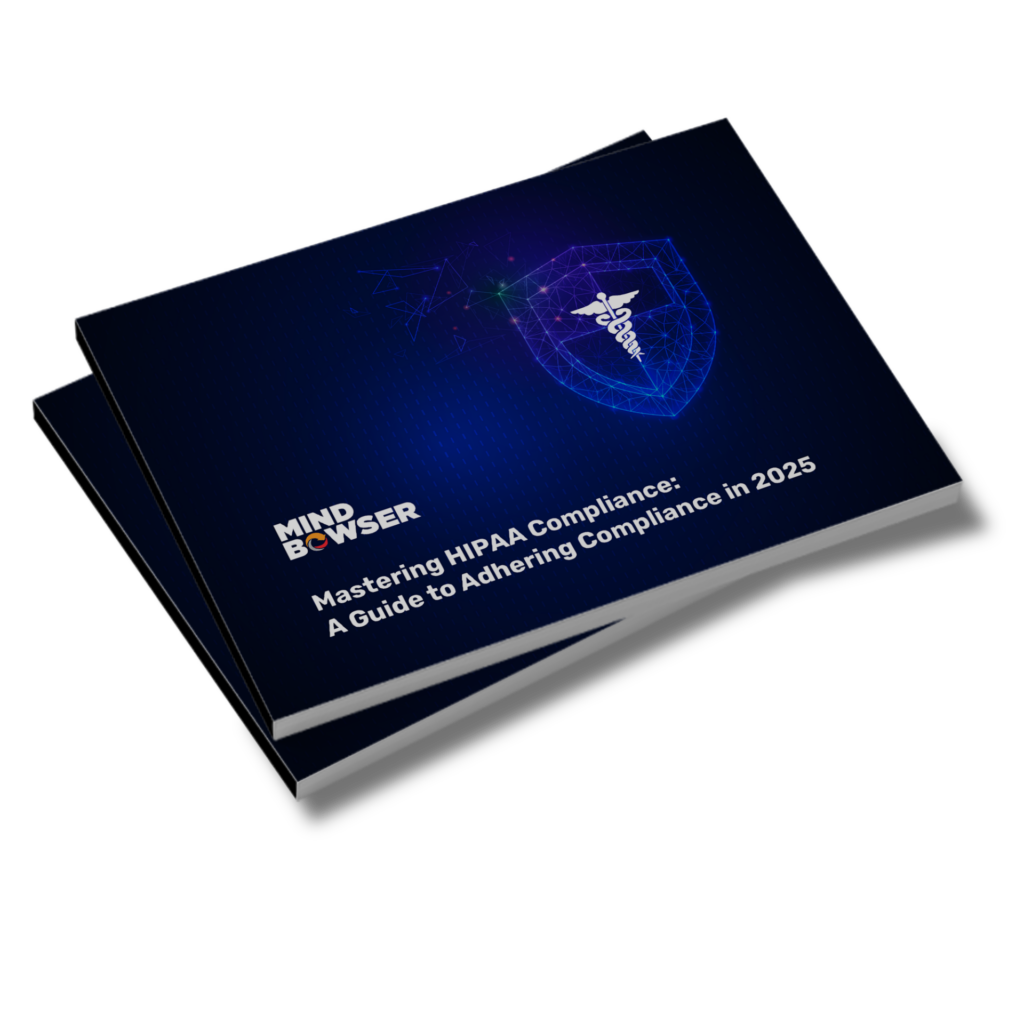
Complete Guide for Complying with HIPAA Regulations
This guide aims to provide you with an understanding of the alterations that have occurred in HIPAA regulations as a result of the COVID-19 pandemic.
Key Requirements for a HIPAA-Compliant App
To build a HIPAA-compliant app, you need to address several important areas, including data encryption, secure storage, access control, and auditing. Here’s how you can implement these in Flutter.
1️⃣ Data Encryption
All PHI must be encrypted when stored (at rest) and during transmission (in transit). This includes encrypting databases, files, and communication between the app and servers.
In Flutter, you can use libraries like `flutter_secure_storage` for storing sensitive data securely on the device. For data transmission, use HTTPS with SSL/TLS encryption.
// Example of secure storage in Flutter
final secureStorage = FlutterSecureStorage();
await secureStorage.write(key: 'token', value: 'secureData');
2️⃣ Authentication and Access Control
Ensure that only authorized users can access patient data. Implement strong authentication methods like multi-factor authentication (MFA) or biometric authentication (fingerprint, face recognition).
You can integrate authentication services like Firebase Authentication, which supports secure login methods including MFA.
3️⃣ User Roles and Permissions
In healthcare apps, different users (patients, doctors, admin) require different levels of access to data. It's essential to define roles and permissions to restrict access to PHI based on user roles.
Use role-based access control (RBAC) to ensure that users can only view or edit data relevant to their role.
4️⃣ Audit Logging
HIPAA requires that any access or modification of PHI is logged. This helps track unauthorised access and provides an audit trail in case of security incidents.
In Flutter, you can log user actions to a secure database like Firebase Firestore or another compliant cloud solution.
5️⃣ Data Backup and Recovery
Regular data backups are mandatory under HIPAA to ensure data can be recovered in case of system failure. Use cloud solutions with automated backup processes, ensuring they are encrypted and compliant.
Schedule a free consultation with our healthcare app experts!
Flutter-Specific Considerations for HIPAA Compliance
Flutter provides powerful tools to develop mobile and web applications, but there are a few specific considerations when aiming for HIPAA compliance:
1️⃣ Using Secure Libraries
Flutter offers a range of libraries and packages to handle sensitive information securely. For example:
- `flutter_secure_storage`: A great option for securely storing sensitive information on devices.
- `Dio` or `HTTP`: For secure network communication, you can use these libraries with SSL/TLS to encrypt data during transmission.
2️⃣ Choosing HIPAA-Compliant Cloud Services
When storing or processing PHI in the cloud, it’s essential to choose cloud services that are HIPAA-compliant. Both Firebase (Google Cloud) and AWS provide HIPAA-compliant services, but you must configure them properly and ensure a Business Associate Agreement (BAA) is in place.
For instance, you can use Firebase services (like Firestore or Realtime Database) for storing non-sensitive data, but for ePHI, ensure encryption is enabled, and you’re following best practices for securing access.
3️⃣ Secure Video and Messaging
If your app involves video consultations or chat functionalities, you need to ensure those are secure. Use a HIPAA-compliant video SDK like Agora or Zoom (with proper configuration for healthcare). Always use end-to-end encryption for video and chat data.
4️⃣ HIPAA-Compliant APIs
Ensure that any third-party APIs you integrate are HIPAA-compliant. This includes APIs for handling patient records, medical images, or insurance data. A BAA may be needed with these services as well.
Related Read: The Secret Weapon of HIPAA Compliance; Business Associate Agreements
Example: Securing a Flutter App for HIPAA Compliance
Below is a simple example showing how to secure PHI in a Flutter app using encrypted storage and HTTPS for secure data transmission:
import 'package:flutter_secure_storage/flutter_secure_storage.dart';
import 'package:http/http.dart' as http;
final secureStorage = FlutterSecureStorage();
// Storing sensitive data securely on the device
await secureStorage.write(key: 'authToken', value: 'yourAuthToken');
// Sending data securely over HTTPS
Future sendDataToServer(String data) async {
final response = await http.post(
Uri.parse('https://your-secure-api.com/sendData'),
headers: {'Authorization': 'Bearer yourAuthToken'},
body: {'data': data},
);
if (response.statusCode == 200) {
print('Data sent successfully');
} else {
print('Failed to send data');
}
}
In this example
- We use `flutter_secure_storage` to store sensitive information like an authentication token securely on the device.
- We ensure that any data sent to the server is over HTTPS, which encrypts the data during transmission.
HIPAA Compliance Made Easy: Protect Patient Privacy and Avoid Costly Fines
This video gives you everything you need to know about HIPAA compliance in healthcare. Learn what qualifies as protected health information (PHI) and how to keep it secure. Understand the key HIPAA rules and avoid hefty fines by following the right practices. Click to watch and ensure your healthcare organization is HIPAA compliant!
Conclusion
Building a HIPAA-compliant healthcare app with Flutter requires careful attention to security, privacy, and regulatory requirements. By implementing encryption, secure storage, authentication, access control, and logging, you can meet the standards set by HIPAA while delivering a seamless user experience.
At Mindbowser, we specialize in developing secure and scalable healthcare applications. With our expertise in Flutter and a deep understanding of HIPAA compliance, we can help you design and build apps that prioritize patient data security while achieving your business goals.
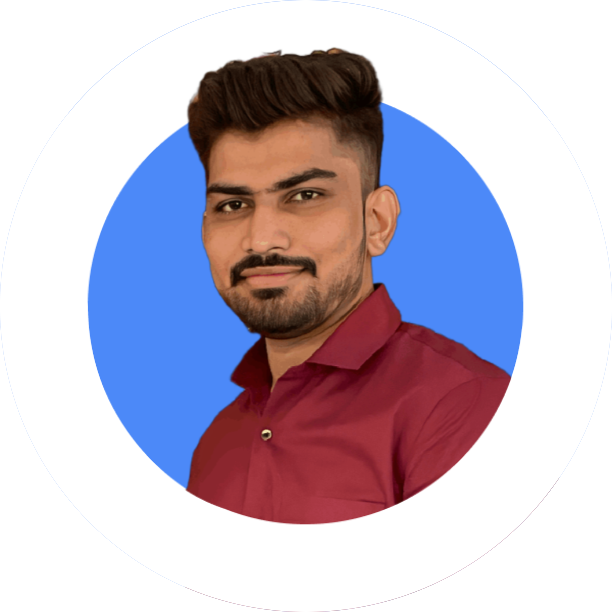
Nikunj Ramani, Flutter Developer
Mobile app developer with over 4 years of experience. I specialize in Flutter, building high-quality, cross-platform mobile applications that deliver seamless user experiences. My expertise spans the entire app development lifecycle, from concept to deployment, ensuring efficient and scalable solutions for diverse projects.
Frequently Asked Questions
- Is Flutter suitable for developing HIPAA-compliant apps?
Yes, Flutter is suitable for building HIPAA-compliant apps. While Flutter provides the tools for secure app development, compliance depends on how you handle PHI, such as using secure storage, encrypting data, implementing user authentication, and integrating HIPAA-compliant backend services.
- How do I ensure secure data storage in a Flutter app?
For secure data storage in Flutter, you can use libraries like flutter_secure_storage to store sensitive data such as authentication tokens. This library encrypts data at rest. For backend storage, ensure that your database is encrypted and HIPAA-compliant.
- What are the penalties for not being HIPAA-compliant?
Non-compliance with HIPAA can lead to significant penalties, ranging from $100 to $50,000 per violation, depending on the level of negligence. Severe violations can also result in criminal charges and reputational damage.
- Does Mindbowser have experience in building HIPAA-compliant healthcare apps?
Yes, at Mindbowser, we have extensive experience in building HIPAA-compliant apps for healthcare clients. Our team understands the nuances of regulatory requirements and uses best practices to ensure that your app adheres to HIPAA standards while delivering a seamless user experience.
Let's Get In Touch
One thing that really stood out to me is the culture and values of the Mindbowser team.
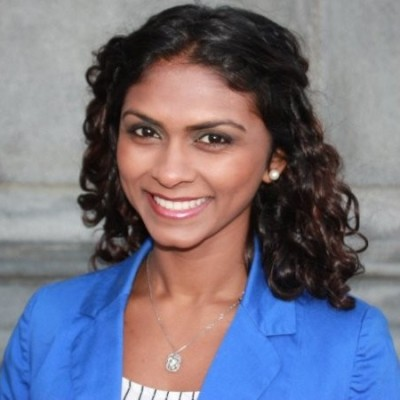
Sanji Silva
Chief Product Officer, Mocingbird
I am so glad I worked with Mindbowser to develop such an Impactful Mobile app.
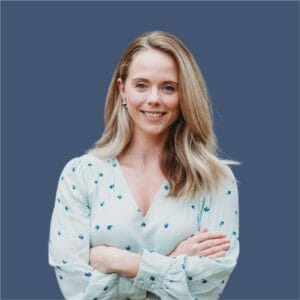
Katie Taylor
Founder and CEO, Child Life On Call
Mindbowser was an excellent partner in developing my fitness app.
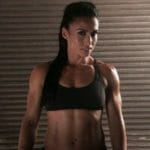
Jirina Harastova
Founder, Phalanx Ubiquity
Mindbowser built both iOS and Android apps for Mindworks, that have stood the test of time. 5 years later they still function quite beautifully. Their team always met their objectives and I'm very happy with the end result. Thank you!
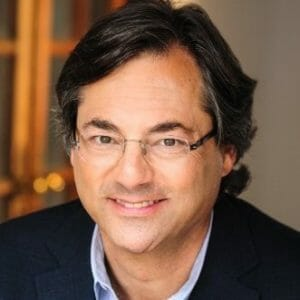
Bart Mendel
Founder, Mindworks
Some of the features conceived, implemented, and designed by the Mindbowser staff are amongst our most popular features.
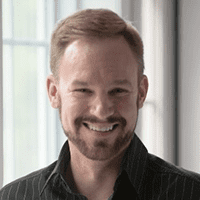
Matthew Amsden
CEO, Proofpilot
Mindbowser is one of the reasons that our app is successful. These guys have been a great team.
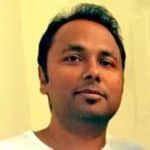
Dave Dubier
Founder & CEO, MangoMirror
The Mindbowser team's professionalism consistently impressed me. Their commitment to quality shone through in every aspect of the project. They truly went the extra mile, ensuring they understood our needs perfectly and were always willing to invest the time to deliver exactly what we envisioned.
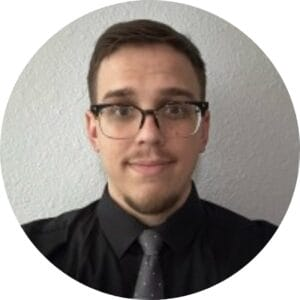
Spencer Barns
Chief Technology Officer, New Day Therapeutics
Kudos for all your hard work and diligence on the Telehealth platform project. You made it possible.
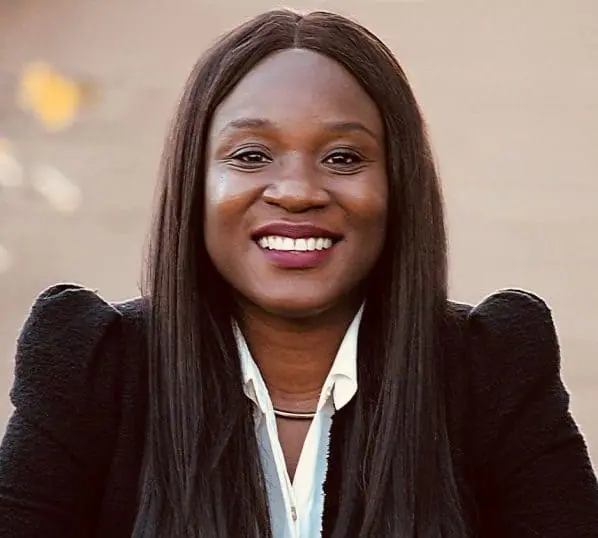
Joyce Nwatuobi
CEO, ThriveHealth